How to Send a SEPA Payment in Javascript
Learn how to send a SEPA Credit Transfer programmatically from any bank in 30 minutes using the ISO20022 standard.
SEPA (Single Euro Payments Area) is the standard for sending bank transfers between companies in the European Union. SEPA payments are sent using the ISO20022 standard, which means that you can use iso20022.js to send SEPA payments programatically.
If you have a corporate bank account within the SEPA area, you can send SEPA payments through your bank. In order for a SEPA payment to be sent, we need to collect the right information about our bank account, the party we're sending money to, and finally create an ISO20022 file and send it to our bank.
Step 1: Understand How Your Bank Accepts SEPA Payments
When you create a SEPA payment, you will need to find a way to deliver the payment to your bank. There are two main ways to send SEPA payments:
1. Upload Payment File To Bank Portal
The easiest way to send SEPA payments is to upload an ISO20022 XML file to your bank's portal. Many banks support this method of sending SEPA payments with their in-house bank portals.
2. Direct Transmission
Direct transmission is the process of sending and receiving payment instructions with your bank. The most common method and reliable interface for direct transmission is through SFTP. This means that the bank will need to provide you with a SFTP server and credentials to connect to it. Think of it as a shared folder where you will deposit instructions into.
Both of these are possible to do using Fiat Web Services, which we will go over at the end of the tutorial.
Step 2: Retrieve Credentials From Your Bank
To send a SEPA payment, you must first have certain core information from your bank. This includes:
- Your bank credentials (Name)
- Your bank account's account number
- Your bank's BIC and country of origin
Using iso20022.js, you might save this information like this:
import { ISO20022 } from 'iso20022.js'
const iso20022 = new ISO20022({
initiatingParty: {
name: 'Iberian Group S.A.',
account: {
iban: 'ES9121000418450200051332'
},
agent: {
bic: 'BSCHESMMXXX',
}
}
});
Step 3: Collect the Creditor (Payee) Party Information
After you know your account information and have the credentials necessary to communicate with your bank, you need to know who are you sending money to, along with some basic information about them. This includes table stakes information about payee such as:
- Creditor's Name (Example: Fjord Dynamics AS)
- Creditor's IBAN (Example: NO93800111111111)
- Creditor's Bank's BIC (Example: NORZNOZZ77)
- Amount in Euros to transfer (Example: 1,000,000 Euros)
In code, you might save the creditor's information like this:
import { Creditor } from 'iso20022.js'
const creditor = {
name: 'Fjord Dynamics AS',
account: {
accountNumber: 'NO93800111111111'
},
agent: {
bic: 'NORZNOZZ77'
},
} as Party
Step 4: Create ISO20022 Payment Initiation Message
You have collected all the prerequisities you need to create an ISO20022-compliant SEPA payment initiation message. ISO20022 is an XML standard, which means it's a machine readable message format that will be able to be programatically processed. SEPA has done the standardization work on our behalf, which means that this standard should be accepted by most banks in Europe.
iso20022.js is the most popular open-source library that you can use to create ISO20022 messages programatically. Using the information you've collected above, you can structure an ISO20022 payment message and bring it to any bank in Europe.
See an interactive demo of how this payment initiation might work in the real world.
Here's how easy it is to create an ISO20022 payment initiation message in one block of code:
const sepa = iso20022.createSEPACreditPaymentInitiation({
paymentInstructions: [{
type: 'sepa',
direction: 'credit',
amount: 1000000, // 10000.00 Euros
currency: 'EUR',
creditor: creditor,
}]
});
fws.paymentTransfers.create(sepa);
Step 5: Send ISO20022 Payment Initiation Message to the Bank
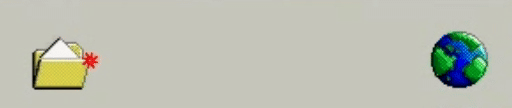
You can save this SEPA payment initiation in Fiat Web Services, which was designed to work with iso20022.js. From there, you can upload the payment file to your bank's portal or programatically send the payment via SFTP.
Retrospective
You have successfully generated a valid SEPA payment instruction. Now you must send the payment to your bank.
You have either of two options:
1. Upload Payment File To Bank Portal
2. Direct Transmission
If you're creating payments at scale for your business, you will eventually need to programatically send payments to your bank. We recommend taking a thoughtful approach to this engineering buildout. First, consider the infrequent manual work of uploading these payments yourself. Then, work with your bank to enable programmatic transmission.
The most common way this can be done is through the use of SFTP. See more about how to send this SFTP file here and consider talking to us here if you need additional resources.
Congrats on sending an SEPA payment over the internet.
If you need to create SEPA payments programatically at scale, you can use iso20022.js to send a SEPA payment in three lines of code. Take a look at our quickstart guide here. From there, you may need to process incoming transactions, view bank balances, and other bank related information in order to run your business.
If you're interacting with the banking system programatically, we want to talk to you.
Click here to schedule a short call with us, and we'd love to get in touch.