How to How to Send a SWIFT Wire in Javascript
Learn how to send a SWIFT wire programmatically from any bank in 30 minutes using the ISO20022 standard.
SWIFT is the most widely used international payment method in the world. In this tutorial we will be going over how to send a SWIFT wire programmatically to your bank, what information you might need about your recipient to allow the payment to clear successfully, and the software you might need to do so. The following example can be implemented in code using iso20022.js.
In order for a SWIFT payment to be sent, we need to collect the right information about our bank account, the party we're sending money to, and finally create an ISO20022 file and send it to our bank.
Step 1: Configure Direct Transmission or File Upload With Your Bank
A company sending SWIFT payments directly must send them through a bank that they operate a Direct Debit Account, or checking account with. In order to communicate with your bank programatically, you will need to configure the bank to allow for direct transmission. This is a process that your bank will need to complete with you.
Direct transmission is the process of sending and receiving payment instructions with your bank. The most common method and reliable interface for direct transmission is through SFTP. This means that the bank will need to provide you with a SFTP server and credentials to connect to it. Think of it as a shared folder where you will deposit instructions into.
This is possible to do using Fiat Web Services, which we will go over at the end of the tutorial.
Step 2: Retrieve Credentials From Your Bank
One of the most important parts of a SWIFT payment initiation is designating the originating information. The SWIFT network needs to understand who the money is coming from. To send SWIFT payments, you need the following information from your bank:
- Your bank's SFTP Host Credentials (URL, Username, Password, ID)
- Your bank account's account number
- Your bank's BIC and country of origin
Eventually we'll be sending an instruction to the bank. Your SFTP credentials will be used to authenticate into the bank's servers. The rest of the information will be in the instruction we send to the bank.
In code, you might save the bank's SFTP credentials as environment variables in a file like this:
SFTP_HOST=sftp.bofa.com SFTP_USERNAME=USERNAME SFTP_PASSWORD=ACME_PASSWORD BANK_UNIQUE_ID=ACMECORP
Using iso20022.js, you might save this information like this:
import { ISO20022 } from 'iso20022.js'
const iso20022 = new ISO20022({
initiatingParty: {
name: 'Acme Corporation',
id: process.env.BANK_UNIQUE_ID,
account: {
accountNumber: '123456789012',
},
agent: {
bic: 'CHASUS33',
bankAddress: {
country: 'US',
}
}
}
});
Step 3: Collect the Creditor (Payee) Party Information
After you know your account information and have the credentials necessary to communicate with your bank, you need to know who are you sending money to, along with some basic information about them. This includes table stakes information about payee such as:
- Creditor's Name (Example: Sakura Technologies)
- Creditor's Bank Account Number (Example: 1234567)
- Creditor's Bank's BIC (Example: BOTKJPJT)
- Creditor's Address (Example: 4-6-16 Ginza, Chuo-ku, Tokyo 104-0061, Japan)
- Amount of JPY to transfer (Example: 1,000,000 JPY)
- Purpose / Remittance Information (Example: Payment for services rendered)
Note that every country has slightly different requirements for the information you need to provide about the payee, usually in the form of a remittance reason or purpose code.
In code, you might save the creditor's information like this:
import { Creditor } from 'iso20022.js'
const creditor = {
name: 'Sakura Technologies',
account: {
accountNumber: '0001001112345'
},
agent: {
bic: 'BOTKJPJT',
},
address: {
streetName: "4-6-16 Ginza",
townName: "Chuo-ku",
postalCode: "104-0061",
country: "JP",
}
} as Creditor
Step 4: Create ISO20022 Payment Initiation Message
You have collected all the prerequisities you need to create an ISO20022-compliant SWIFT payment initiation message. ISO20022 is an XML standard, which means it's a machine readable message format that will be able to be programatically processed. SWIFT has done the standardization work on our behalf, which means that this standard should be accepted by most banks in the world.
iso20022.js is the most popular open-source library that you can use to create ISO20022 messages programatically. Using the information you've collected above, you can structure an ISO20022 payment message and bring it to any bank in the world.
See an interactive demo of how this payment initiation might work in the real world.
Here's how easy it is to create an ISO20022 payment initiation message in one block of code:
const creditPaymentInitiation = iso20022.createSWIFTCreditPaymentInitiation({
paymentInstructions: [{
type: 'swift',
direction: 'credit',
amount: 1000000,
currency: 'JPY',
creditor: creditor,
remittanceInformation: 'Payment for services rendered'
}]
});
Step 5: Send ISO20022 Payment Initiation Message to the Bank
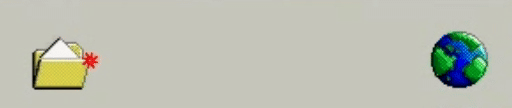
You can save this SWIFT payment initiation in Fiat Web Services, which was designed to work with iso20022.js. From there, you can upload the payment file to your bank's portal or programatically send the payment via SFTP.
Retrospective
Congrats on sending an international SWIFT payment over the internet.
If you need to create SWIFT payments programatically, you can use iso20022.js to send a SWIFT payment in three lines of code. Take a look at our quickstart guide here. From there, you may need to process incoming transactions, view bank balances, and other bank related information in order to run your business.
If you're interacting with the banking system programatically, we want to talk to you.
Click here to schedule a short call with us, and we'd love to get in touch.